In our previous articles, we have learned about the basic programming concepts of Python. In this article, let us use those concepts to write some basic Python programs. This will gives a good practice of what we have learned so far in Python.
Table of Contents
Print Hello World in Python
print("Hello World") #this line is used to print
input() #used to hold screen
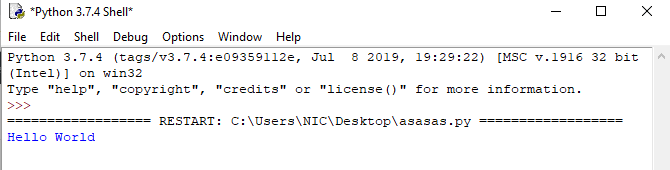
Addition of two Number In Python
num1=int(input("Enter the First Number "))
num2=int(input("Enter the Second Number "))
num3=num1+num2
print("The Sum is = ",num3)
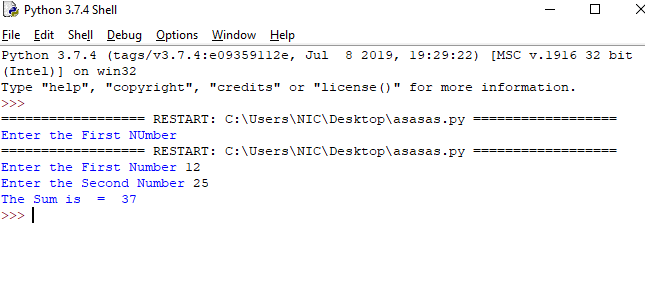
Find Area of Circle in Python
r=float(input("Enter the Radious of circle "))
area=3.14*r*r
print("Area of Circle = ",area)
input("Press any key to exit")
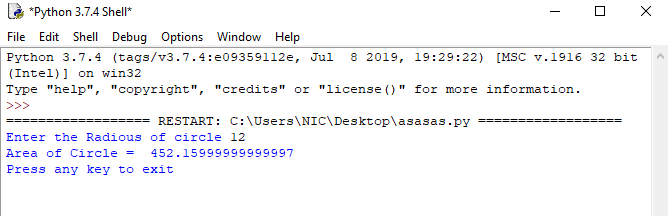
Find Area of Rectangle in Python
length=int(input("Enter the Length of Ractangle "))
breadth=int(input("Enter the Breadth of Ractangle "))
area=length*breadth
print("Area of Ractange is = ",area)
input("Press any key to Exit")
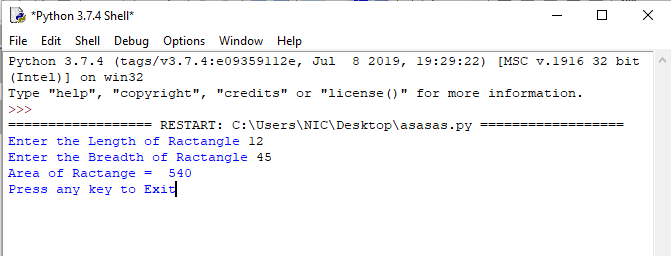
Python Program to Create the Salary Slip in Python
id=input("Enter the Employee Id : ")
name=input("Enter the Name of Employee : ")
dsg=input("Enter the Designation : ")
basic=int(input("Enter the Basic Salary: "))
l=int(input("Enter the Total Leaves : "))
lamount=basic/30*l
ta=basic*5/100
da=basic*6/100
hra=basic*7/100
ma=3500
gross=basic+ta+da+hra+ma
itax=basic*5/100
net=gross-itax-lamount
print("---------Salary Slip----------")
print("Employee Id : ",id)
print("Name : ",name)
print("Designation : ",dsg)
print("------------------------------")
print("Leaves : ",l)
print("Leaves Amount : ",lamount)
print("------------------------------")
print("Ta\tDa\tHra\tMa")
print(ta,"\t",da,"\t",hra,"\t",ma)
print("------------------------------")
print("Gross Salary : ",gross)
print("Income Tax : ",itax)
print("Net Salary : ",net)
print("------------------------------")
input()
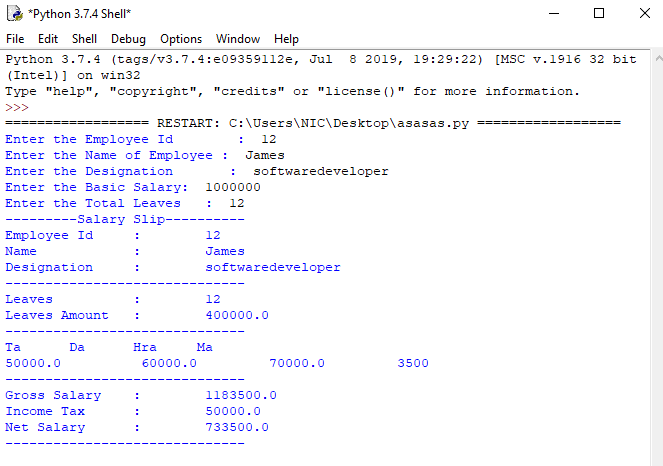
Python program to calculate the simple interest
p=int(input("Enter the Principal Amount "))
rate=float(input("Enter the Rate of Interest "))
time=int(input("Enter the Total Time "))
si=(p*rate*time)/100
print("Simple Interest = ",si)
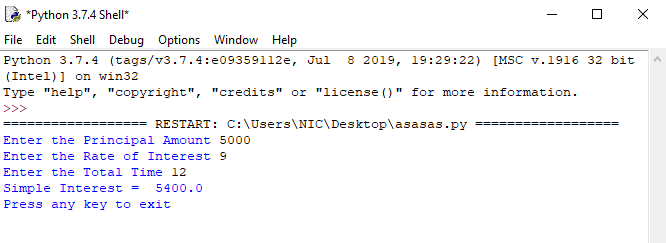
Python Program to calculate the Square and Square root
import math
num=int(input("Enter the Number "))
print("Square of ",num," is ",math.pow(num,2))
print("Square Root of ",num," is ",math.sqrt(num))
print("Cube of ",num," is ",math.pow(num,3))
input()
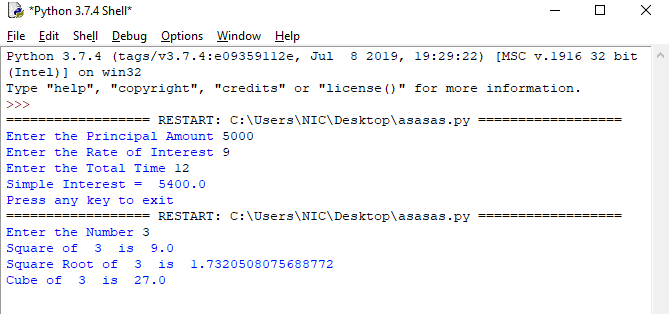