In our previous article, we have learned about the basic concept of List in python. we have learned about lots of operations we can do with List. In this article, let us use the concepts we have learned so far and write some programs on Python Lists.
Table of Contents
Python Program to Add the element in List
# append(): add an obj in list at last position
a=[10,20,30,40,50,60]
print("Original List is ",a)
a.append(70) # adding the Element in the list
print("Appended List is ",a)
input()
Python program to Print the list item
shoping_list=["Shirt","Pent","Shoes"]
print(shoping_list[0]) # Using the index value to print the element
print(shoping_list[2]) # Using the index value to print the element
input()
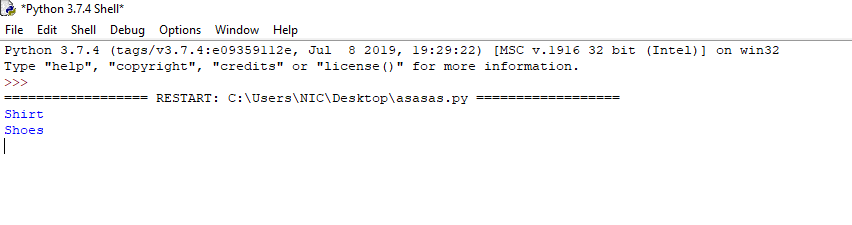
Python Program to calculate the occurrence of any element in the list
#count(): used to calculate occurance of any element in list
a=[10,20,30,40,50,10,10,10,20]
n=int(input("Enter the Number to count in list "))
print(n," Exist ",a.count(n)," times in list")
input()
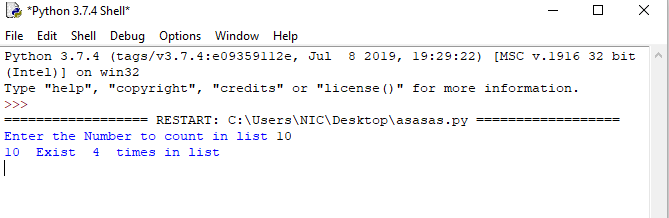
Python Program to Delete the Element from the List
# del is used to delete an obj from any given position from list
a=[10,20,30,40,50,60]
print("Original List is ",a)
del a[2]
print("List After Deletion is ",a)
input()
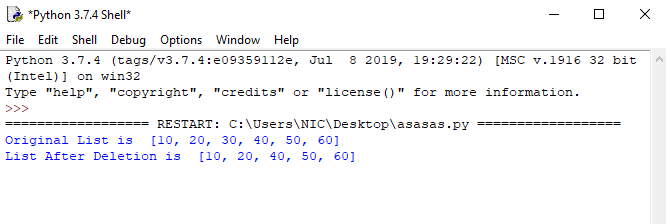
Python Program to Delete the Element using other methods
#all three used to delete any element from list
#pop()
#del
#remove()
a=[10,20,30,40,50,60,70,80,60,90,100]
print("Original List is a =\n",a)
del a[4]
print("List after Deleting 4th index value is a =\n",a)
a.pop()
print("List after pop method is a =\n",a)
a.pop(1)
print("List after pop(index) method is a =\n",a)
a.remove(60)
print("List after remove(element) method is a =\n",a)
input()
Python Program to Update the LIst
#updating a list
shoping_list=["Shirt","Pent","Shoes"]
print(shoping_list)
shoping_list[1]="Belt"
print(shoping_list)
input()
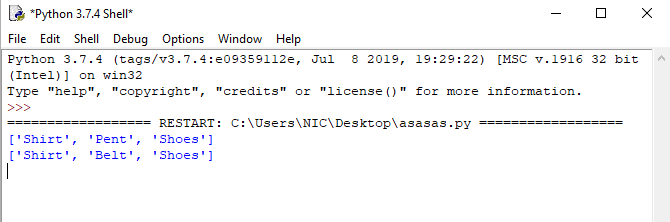
Python program to add two list
#extend(): extend a list b to list a
a=[10,20,30,40,50]
b=[1,2,3,4,5]
print("original List a = ",a)
a.extend(b)
print("List a After Extend = ",a)
input()
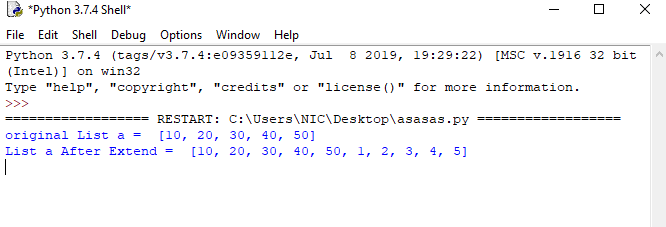
Python Program to calculate the length of List
# len() used to get length of list
a=[1,2,3]
b=[1,2,4,5,8]
print("Length of List a is =",len(a))
print("Length of List a is =",len(b))
input()
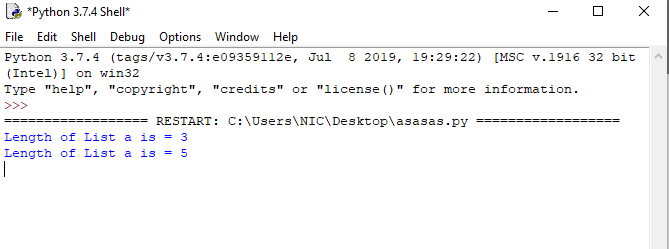
Python Program to calculate the max and min element in List
# min() : is used to get the minium value from the list
#max() : is used to get the maxium value from the list
a=[232,34,4,25,2455,325,343]
print("Smallest Element is = ",min(a))
print("greatest Element is = ",max(a))
input()
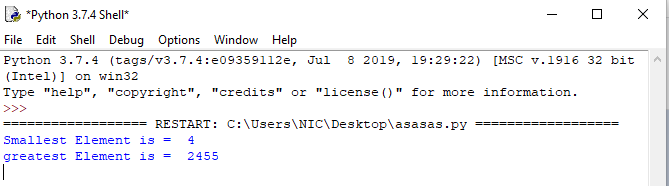
Python program to calculate the sum of a list
#sum(): used to calculate sum of Element of a list
a=[10,20,30,40,50]
sum=0
for i in range(0,len(a)):
sum=sum+a[i]
print("Sum of Elements of list = ",sum)
input()
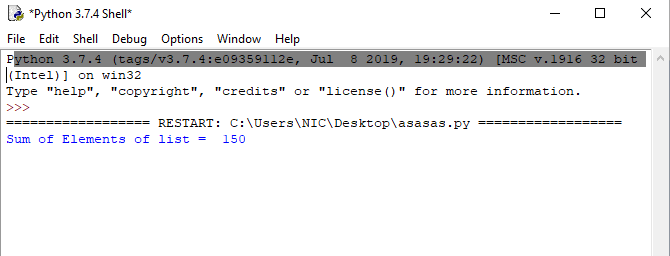
Python program to compare the list without using builtin function
#compate two list
a=[1,2,3]
b=[1,2,3,4]
f=0
i=0
while(i<len(a)):
if(a[i]!=b[i]):
f=1
break
i=i+1
if(f==0 and len(a)==len(b)):
print("Both List are Same")
else:
print("Both List are not same")
input()
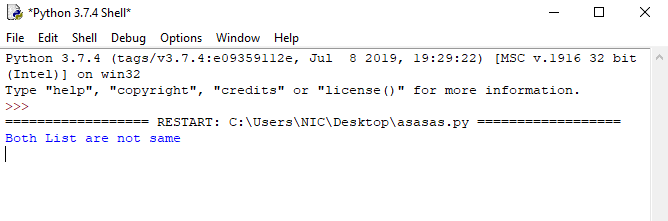
Python Program to Reverse the List
#reverse():used to reverse a list
a=[1,2,3,4,5,6,7,8,9,10]
print("Original List is\n",a)
a.reverse()
print("Reverse List is\n",a)
input()
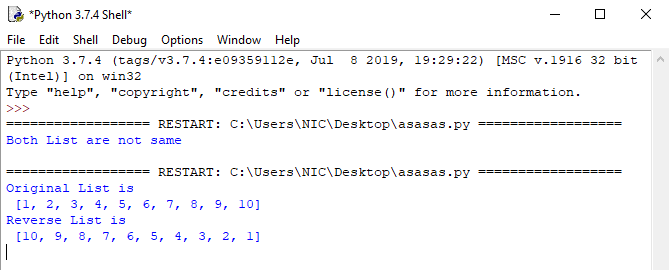
Binary Search in Python
#Searching:
#Binary search
print("Enter Element in List by space\n")
a=[int(x) for x in input().split()]
print("Original List is \n",a)
a.sort()
start=0
end=len(a)-1
mid=int((start+end)/2)
n=int(input("Enter the Number to Search in list "))
while(n!=a[mid] and start<=end):
if(n<a[mid]):
end=mid-1
else:
start=mid+1
mid=int((start+end)/2)
if(n==a[mid]):
print(n," Exist in List at a[",mid,"]")
else:
print(n,"Does not Exist in List")
input()
#Time Complexity of Linear Search = O(n)
#Time COmplexity of Binary Search O(log2n): log(n)/log(2)
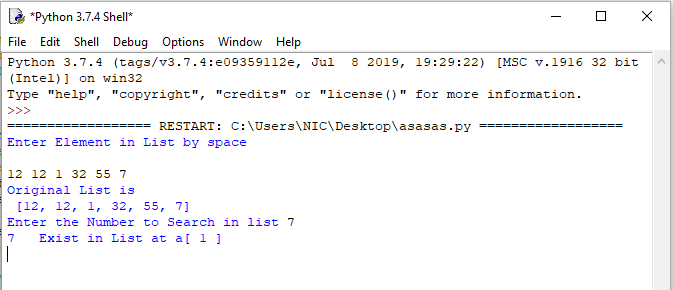
Linear Search in Python using List
#Searching:
#linear search
print("Enter Element in List seperate by space\n")
a=[int(x) for x in input().split()]
c=0
n=int(input("Enter the Number to count in list "))
for i in range(0,len(a)):
if(a[i]==n):
print(n," found at a[",i,"]")
c=c+1
if(c==0):
print(n," Does not Exist in List")
else:
print("Total Result Found = ",c)
input()
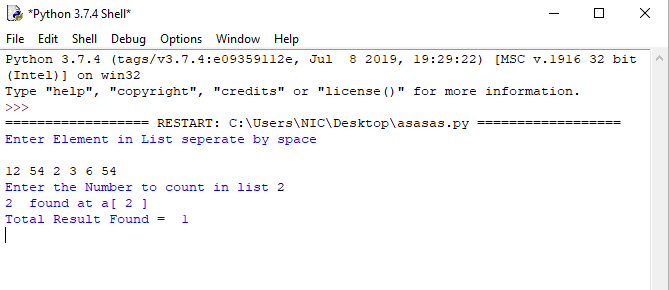
Python Program to short the List in ascending order and descending order
#sort():arrange list in ascending order/Descending order
a=[100,20,80,40,60,50,70,30,90,10]
print("Original List is\n",a)
a.sort(reverse=1)#descending
print("Sorted List is\n",a)
a.sort()#Ascending
print("Sorted List is\n",a)
input()
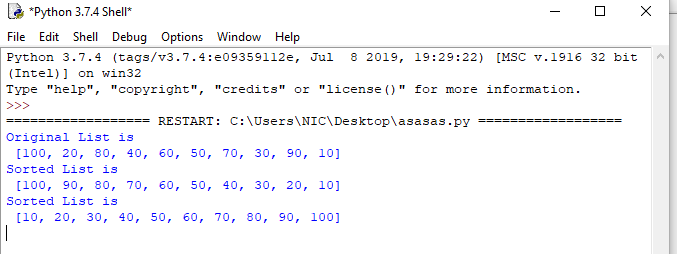
Python program to sort the list by input Element
print("Enter Element in List\n")
a=[int(x) for x in input().split()]
print("Original List is \n",a)
for i in range(0,len(a)-1):
for j in range(i+1,len(a)):
if(a[i]>a[j]):
temp=a[i]
a[i]=a[j]
a[j]=temp
print("Sorted List is \n",a)
input()
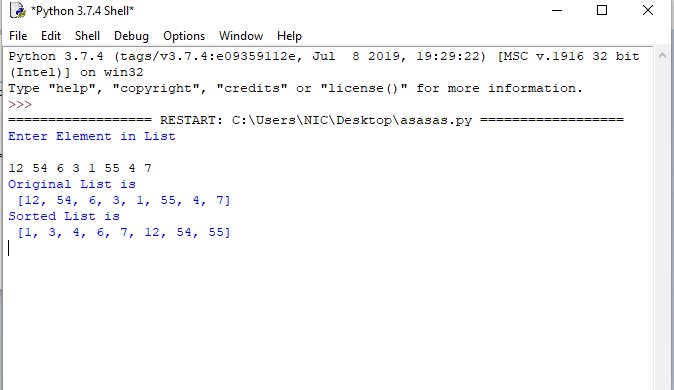