In previous articles, we have learned about string operations in Python. In this article, let us create some basic Python programs on Strings. We will perform some basic operations using the string in Python. I believe this will strengthen your concept of string operations in Python.
Table of Contents
How to Concatenate two String
str1='Hello'
str2="Python"
str3=str1+" "+str2
print("String after Concatination is ",str3)
input()
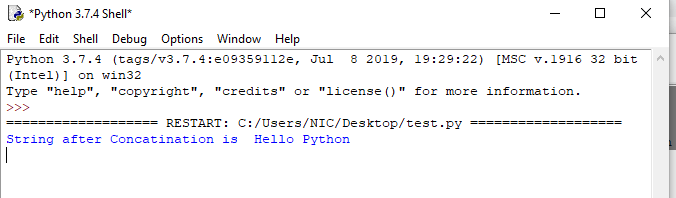
Python program to check whether a string is terminated with any particular suffix or not
#endswith():used to check wheather a string is terminated with any perticular suffix or not
str="Hello World."
print(str.endswith("World"))
print(str.endswith("World."))
print(str.endswith("ld."))
input()
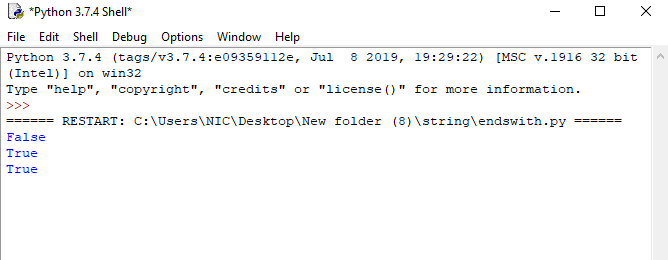
Program to check whether particular character found in string or not
# we are using the in and not in to check
str="Hello Python"
print('Hello' in str)
print('e' in str)
print('M' not in str)
print('H' not in str)
input()
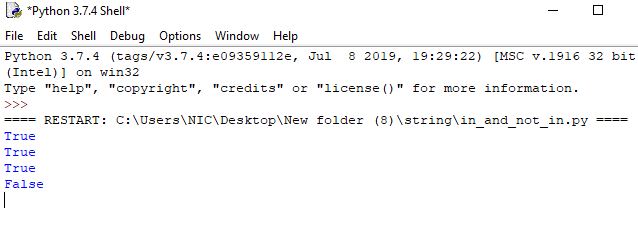
Python program to get the length on the string
#len():- return length of string:
str="Hello World"
print("Lengt of String is = ",len(str))
input()
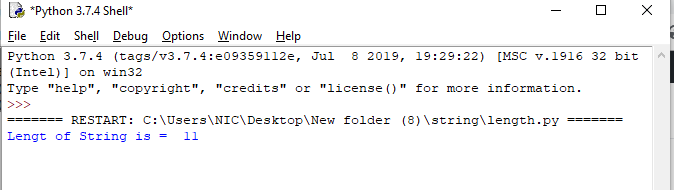
Program to check string have a digit or alphabets
#isdigit():- return ture is string has only digits ortherwise false
#isalpha():=return ture is string has only alphabets ortherwise false
str="12345"
print(str.isdigit())
str="123@#$$45"
print(str.isdigit())
str="Ducat Education"
print(str.isalpha())
str="DucatEducation"
print(str.isalpha())
input()
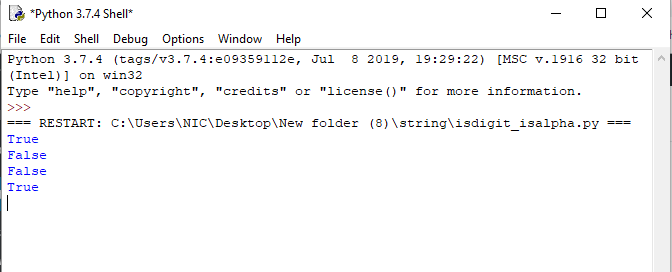
Python Program to return the position of any substring
#index():- return index of any perticular substring
str="Hello World Hello World"
pos=str.index("World")
print("First Position of word is ",pos)
print("Secod Position of World is = ",str.index("World",pos+1))
input()
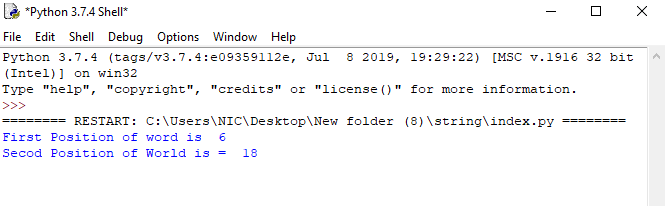
Python Program to check string is palindrome or not
str=input("Enter the String : ")
length=len(str)
print("Original String is = ",str)
s=""
for i in range(0,length):
s=str[i]+s
print("Reversed String is = ",s)
if(str==s):
print("String is Palindomre")
else:
print("Not Palindomre")
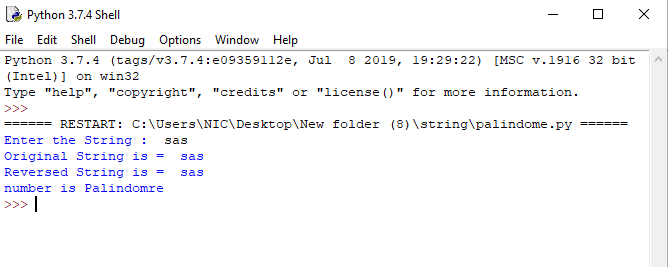
Python program to check substring containing how many palindromes
str=input("Enter the String : ")
length=len(str)
c=0
for i in range(0,length):
for j in range(i+2,length):
temp=""
for k in range(i,j+1):
temp=temp+str[k]
x=len(temp)
f=0
for k in range(0,int(x/2)):
if(temp[k]!=temp[x-1-k]):
f=1
break
if(f==0):
print(temp)
c=c+1
print("Total Palindrome Strings are : ",c)
input()
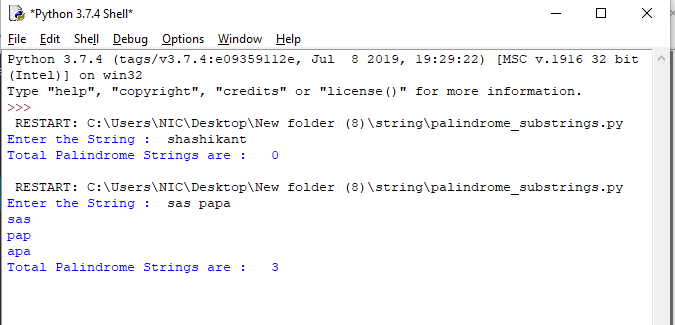
Python program to slice the String
#[:] Range Slice
str="Hello Python"
print(str[0:10])
print(str[1:8])
print(str[2:9])
print(str[3:11])
input()
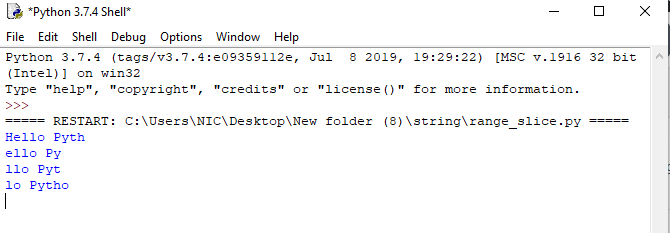
Python program to replace any substring with any particular string
#replace():used to replace any substring of string with any perticular string
str="team Education"
str=str.replace("team","TEAM")
print(str)
input()
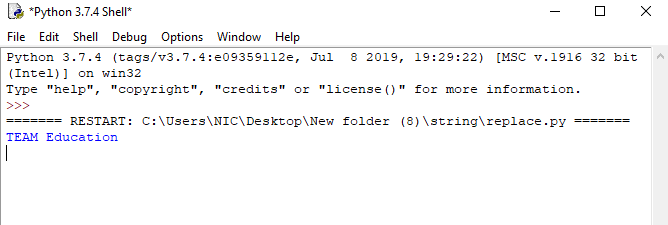
Python program to print the particular string
str="Hello Python"
print(str[2])
input()
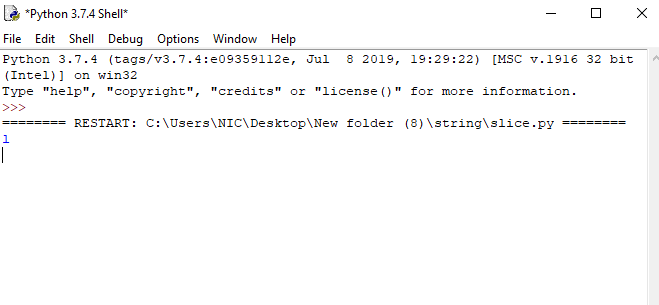
Python program to reverse the string
str=input("Enter the String : ")
length=len(str)
print("Original String is = ",str)
s=""
for i in range(0,length):
s=str[i]+s
print("Reversed String is = ",s)
input()
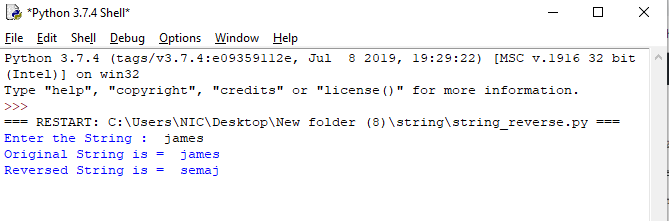